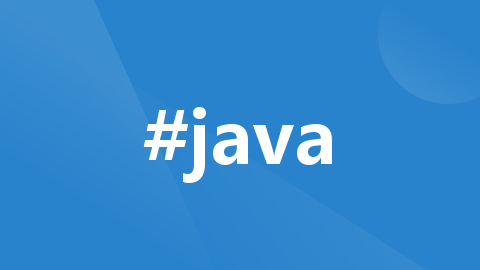
Unity如何接入DeepSeek-R1
建议后续扩展功能:流式响应处理、上下文记忆、本地请求队列管理等。
·
在Unity 2022.3.5中接入DeepSeek R1(类似API服务)的关键步骤如下,以HTTP请求和协程为核心:
Step 1 - 准备工作
-
获取API凭证
- 前往DeepSeek官网注册账号
- 在控制台创建应用并获取
API Key
(通常以sk-
开头)
-
查看API文档
- 确认API端点地址(如
https://api.deepseek.com/v1/chat/completions
) - 记录请求头、请求体格式要求
- 确认API端点地址(如
Step 2 - 创建Unity脚本
using UnityEngine;
using UnityEngine.Networking;
using System.Collections;
using System.Text;
public class DeepSeekIntegration : MonoBehaviour
{
private const string API_URL = "https://api.deepseek.com/v1/chat/completions";
private const string API_KEY = "sk-your-key-here"; // 替换为你的实际密钥
public void SendRequest(string prompt)
{
StartCoroutine(PostRequest(prompt));
}
private IEnumerator PostRequest(string prompt)
{
// 构建请求体
var requestBody = new RequestBody
{
model = "deepseek-r1",
messages = new[]
{
new Message { role = "user", content = prompt }
},
max_tokens = 1000
};
string jsonBody = JsonUtility.ToJson(requestBody);
byte[] bodyRaw = Encoding.UTF8.GetBytes(jsonBody);
// 创建WebRequest
UnityWebRequest request = new UnityWebRequest(API_URL, "POST");
request.uploadHandler = new UploadHandlerRaw(bodyRaw);
request.downloadHandler = new DownloadHandlerBuffer();
request.SetRequestHeader("Content-Type", "application/json");
request.SetRequestHeader("Authorization", $"Bearer {API_KEY}");
// 发送请求
yield return request.SendWebRequest();
// 处理响应
if (request.result == UnityWebRequest.Result.Success)
{
var response = JsonUtility.FromJson<Response>(request.downloadHandler.text);
Debug.Log($"AI回复:{response.choices[0].message.content}");
}
else
{
Debug.LogError($"请求失败:{request.error}");
}
}
// JSON数据结构
[System.Serializable]
public class RequestBody
{
public string model;
public Message[] messages;
public int max_tokens;
}
[System.Serializable]
public class Message
{
public string role;
public string content;
}
[System.Serializable]
public class Response
{
public Choice[] choices;
}
[System.Serializable]
public class Choice
{
public Message message;
}
}
Step 3 - 使用示例
// 在任意脚本中调用
DeepSeekIntegration deepSeek = GetComponent<DeepSeekIntegration>();
deepSeek.SendRequest("用Unity实现角色移动的代码示例");
关键注意事项
-
协程处理
- 必须使用
StartCoroutine
调用异步请求 - 避免在Update等高频方法中直接调用
- 必须使用
-
安全建议
- 正式项目应将API Key存储在服务端
- 客户端建议使用临时Token或代理服务器
-
性能优化
- 添加请求超时处理
request.timeout = 10; // 设置10秒超时
- 使用对象池复用请求实例
-
2022.3.5版本特性
- 使用
UnityWebRequest
替代旧版WWW
- 推荐安装
Newtonsoft Json.NET
包处理复杂JSON
Window > Package Manager > 搜索"Newtonsoft Json" > 安装
- 使用
测试验证
- 在Hierarchy中创建空物体并挂载脚本
- 在Console面板观察请求日志
- 使用简单prompt测试连通性(如"Hello")
- 通过断点检查JSON反序列化是否正确
如遇SSL证书错误
,可添加以下预处理指令:
using UnityEngine.Networking;
// 在Awake方法中插入
#if UNITY_EDITOR
UnityWebRequest.ClearCookieCache();
UnityWebRequest.ClearUrlCache();
UnityEngine.Application.SetStackTraceLogType(LogType.Log, StackTraceLogType.None);
#endif
建议后续扩展功能:流式响应处理、上下文记忆、本地请求队列管理等。
更多推荐
所有评论(0)