使用unity第一次在Android环境开发记录(第一部分)
创建空物体,将空物体改名为Car,右键Transform选择Reset,将汽车模型拖到空物体下方,给汽车模型添加Rigidbody和Box Collider组件,点击Box Collider组件中Edit Collider后面的按钮,拖动碰撞盒的大小和汽车模型相同。在场景中新建一个空物体ScoreDisplay,在ScoreDisplay下面新建一个Canvas画布,画布分辨率与当前手机相同。4.
·
- 导入Input System包,通过鼠标模拟手指滑动屏幕
- Device Simulator Devices包,UI适配神器 移动设备模拟器
- 配置Input System和Device Simulator Devices
4.搭建场景,并将场景中的障碍物标签设置为Obstacle。
5.汽车移动实现
创建空物体,将空物体改名为Car,右键Transform选择Reset,将汽车模型拖到空物体下方,给汽车模型添加Rigidbody和Box Collider组件,点击Box Collider组件中Edit Collider后面的按钮,拖动碰撞盒的大小和汽车模型相同。将Box Collider中的Is Trigger按钮勾选上。
在Car物体下新建一个Car脚本,代码如下:
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class Car : MonoBehaviour
{
[SerializeField] private float speed = 10f;
[SerializeField] private float speedGainPerSecond = 0.2f;
[SerializeField] private float turnSpeed = 200f;
private int steerValue;
void Update()
{
speed += speedGainPerSecond * Time.deltaTime;
transform.Rotate(0, steerValue * turnSpeed * Time.deltaTime, 0);
transform.Translate(Vector3.forward * speed * Time.deltaTime);
}
public void OnTriggerEnter(Collider other)
{
if (other.tag == "Obstacle")
{
SceneManager.LoadScene("Scene_MainMenu");
}
}
public void SetSteerValue(int value)
{
steerValue = value;
}
}
创建一个画布,左右各一半Image图片,将图片透明度设为0.
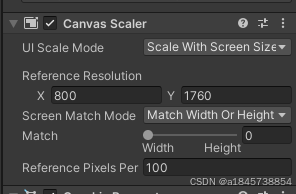
在Left和Right下添加组件
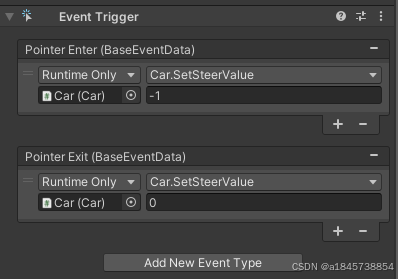
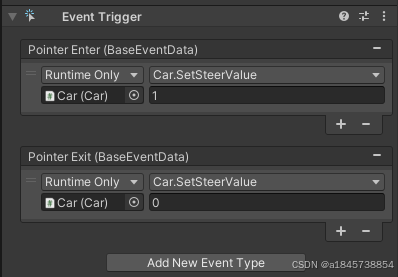
6.设置相机跟随汽车移动
在Hierarchy右键创建虚拟相机
7.得分的显示
在场景中新建一个空物体ScoreDisplay,在ScoreDisplay下面新建一个Canvas画布,画布分辨率与当前手机相同。
在上方场景一个文本显示得分
在ScoreDisplay下新建一个脚本ScoreSystem,代码如下:
using System.Collections;
using System.Collections.Generic;
using TMPro;
using UnityEngine;
// 计分系统类,用于管理游戏中的得分和最高分记录
public class ScoreSystem : MonoBehaviour
{
// 在Inspector中暴露的字段,用于显示得分的文本组件
[SerializeField] private TMP_Text scoreText;
// 在Inspector中暴露的字段,用于设定得分的倍率
[SerializeField] private float scoreMultiplier;
// 成员变量,用于累计得分
private float score;
// 常量,用于存储最高分的键名
public const string HighScoreKey = "HighScore";
// 每帧调用,用于更新得分和显示
void Update()
{
// 根据时间流逝和得分倍率增加得分
score += Time.deltaTime * scoreMultiplier;
// 更新得分显示,将得分值四舍五入后转换为字符串
scoreText.text = Mathf.FloorToInt(score).ToString();
}
// 当对象被销毁时调用,用于保存最高分
void OnDestroy()
{
// 从PlayerPrefs中获取当前的最高分
int highScore = PlayerPrefs.GetInt(HighScoreKey, 0);
// 如果当前得分高于最高分,则更新最高分记录
if(score > highScore)
{
PlayerPrefs.SetInt(HighScoreKey, Mathf.FloorToInt(score));
}
}
}
将得分文本拖入Score Text
保存场景为Scene_Game
更多推荐
所有评论(0)